DLL Operation: Insert After
Suppose we want to insert a node after the one pointed to by the reference variable target
:

Exercise Complete the implementation of insertAfter
.
public void insertAfter(Node<T> target, T data) {
// TODO Implement me!
}
Hint: Use the following visualization as guidance.
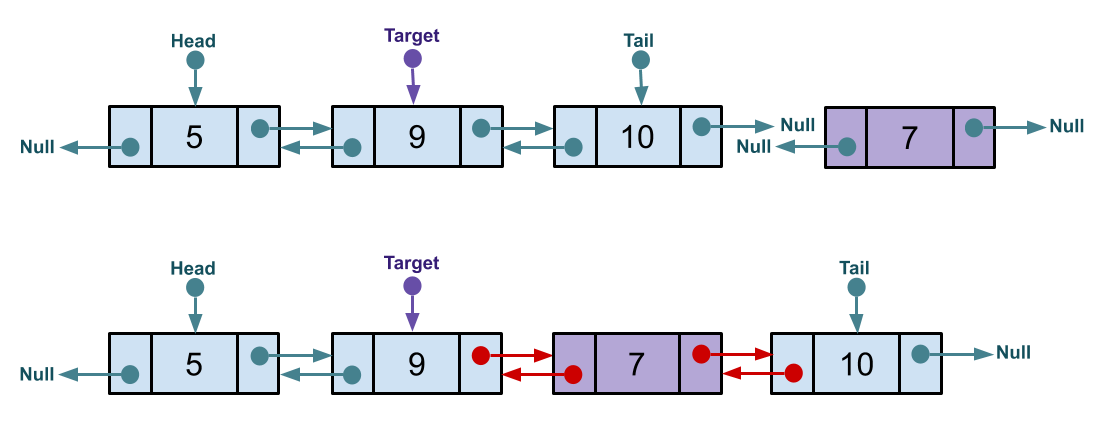
Solution
public void insertAfter(Node<T> target, T data) {
Node<T> nodeToInsert = new Node<>(data);
Node<T> nextNode = target.next;
target.next = nodeToInsert;
nodeToInsert.prev = target;
nodeToInsert.next = nextNode;
nextNode.prev = nodeToInsert;
}
Caution: the implementation above fails to account for edge cases!